数据可视化(Python)
鸢尾花(Iris
)数据集如何利用pandas, matplotlib
和seaborn
库进行可视化分析。
1 | # First, we'll import pandas, a data processing and CSV file I/O library |
让我们看一下数据,结果输出为:
1 | Id SepalLengthCm SepalWidthCm PetalLengthCm PetalWidthCm Species(物种) |
每个物种有多少个实例:
1 | # Let's see how many examples we have of each species |
结果输出为:
1 | Iris-setosa 50 |
我使用pandas
数据帧的.plot()
绘图能力来制作鸢尾花(Iris
)特征的散点图。
1 | # The first way we can plot things is using the .plot extension from Pandas dataframes |
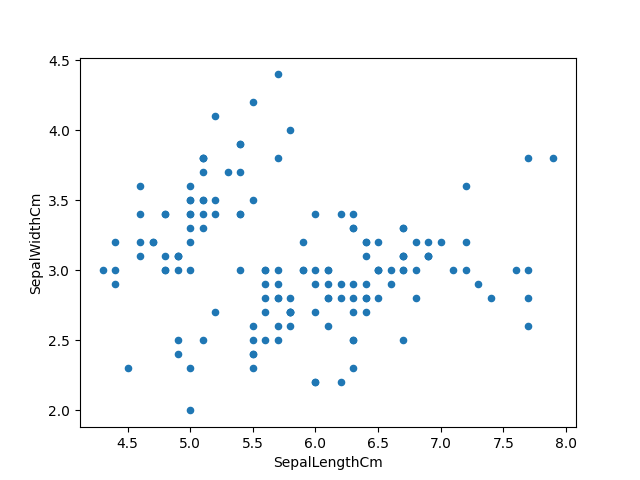
我们还可以使用seaborn
库来制作类似的散点图,Seaborn
组合图在同一图中显示双变量散点图和单变量直方图:
1 | # We can also use the seaborn library to make a similar plot |
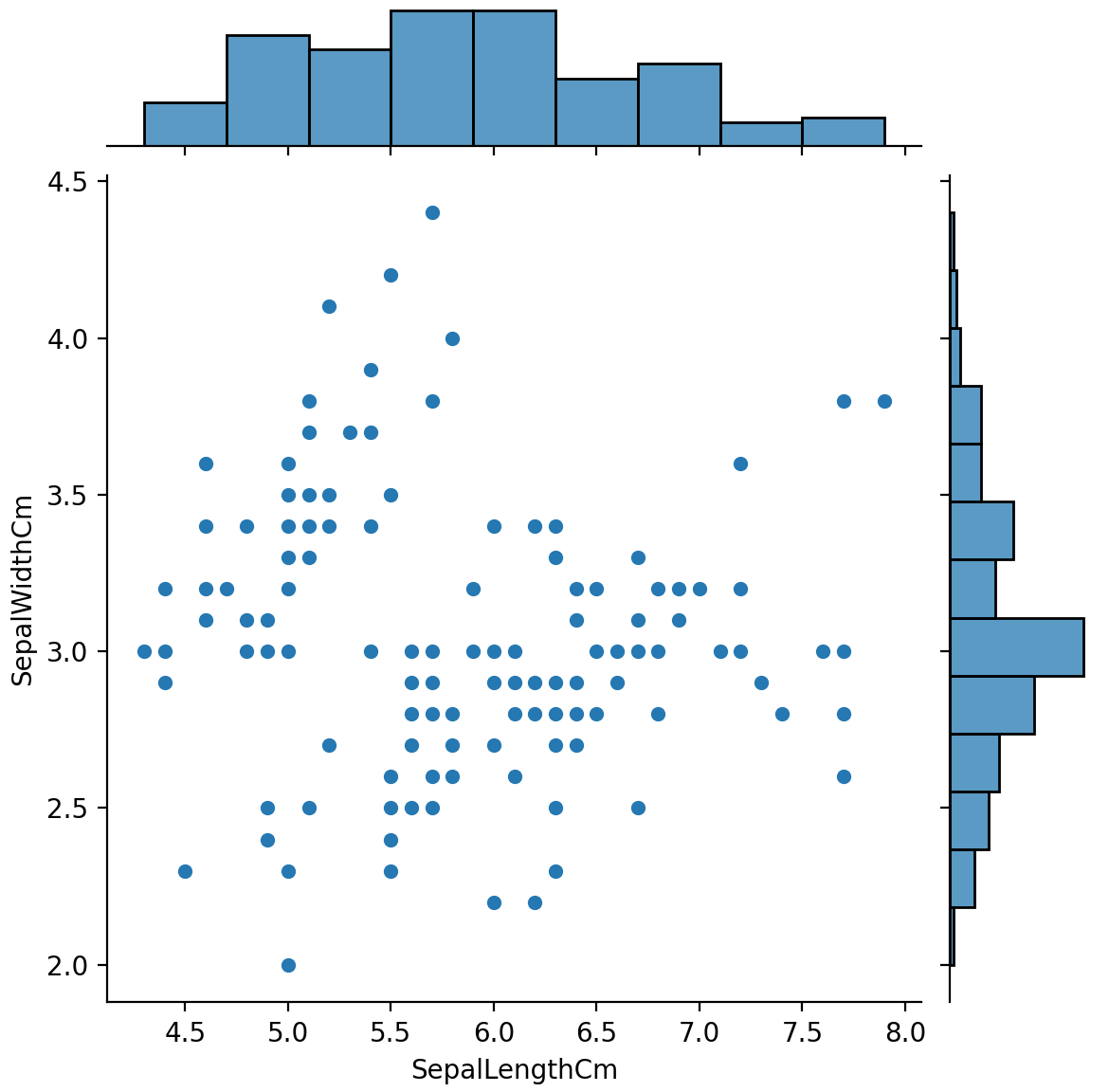
上图中缺少每种植物的物种信息。我们将使用seaborn
的FacetGrid
按物种为散点图着色:
1 | # One piece of information missing in the plots above is what species each plant is |
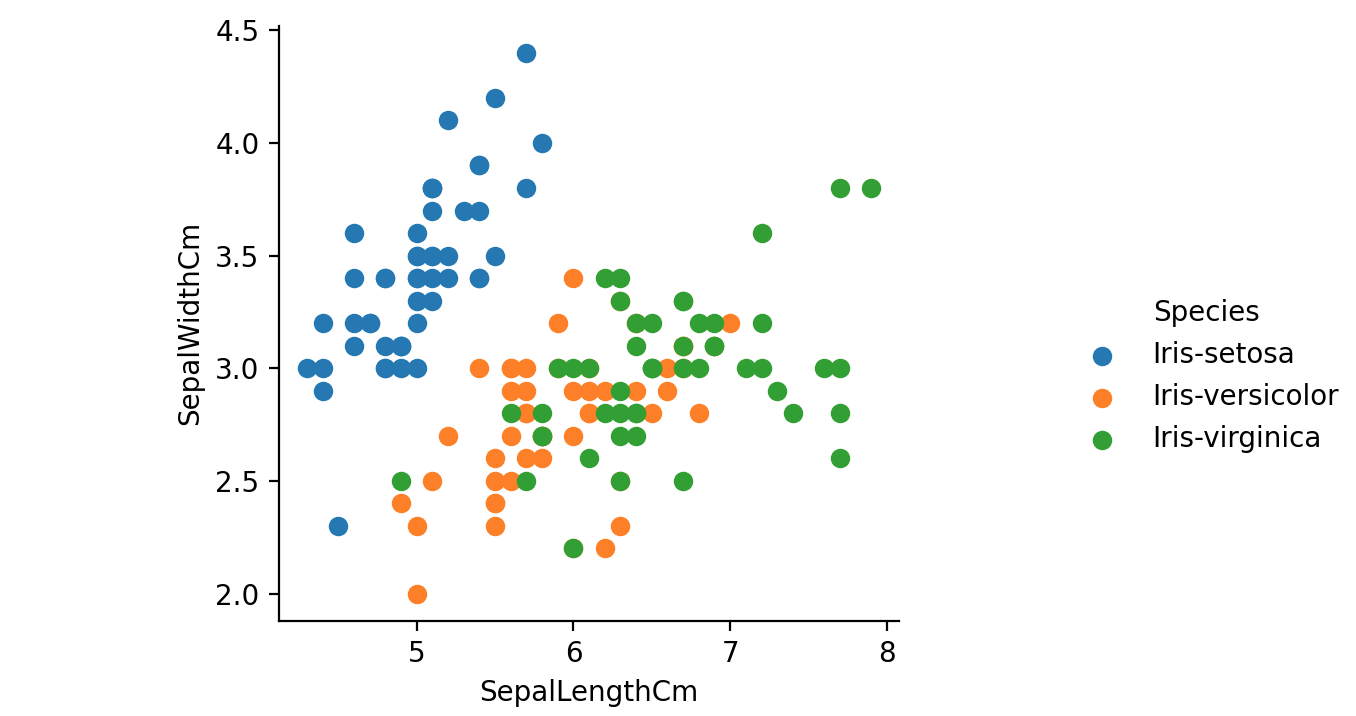
我们可以通过箱线图查看Seaborn
中的单个特征。
1 | # We can look at an individual feature in Seaborn through a boxplot |
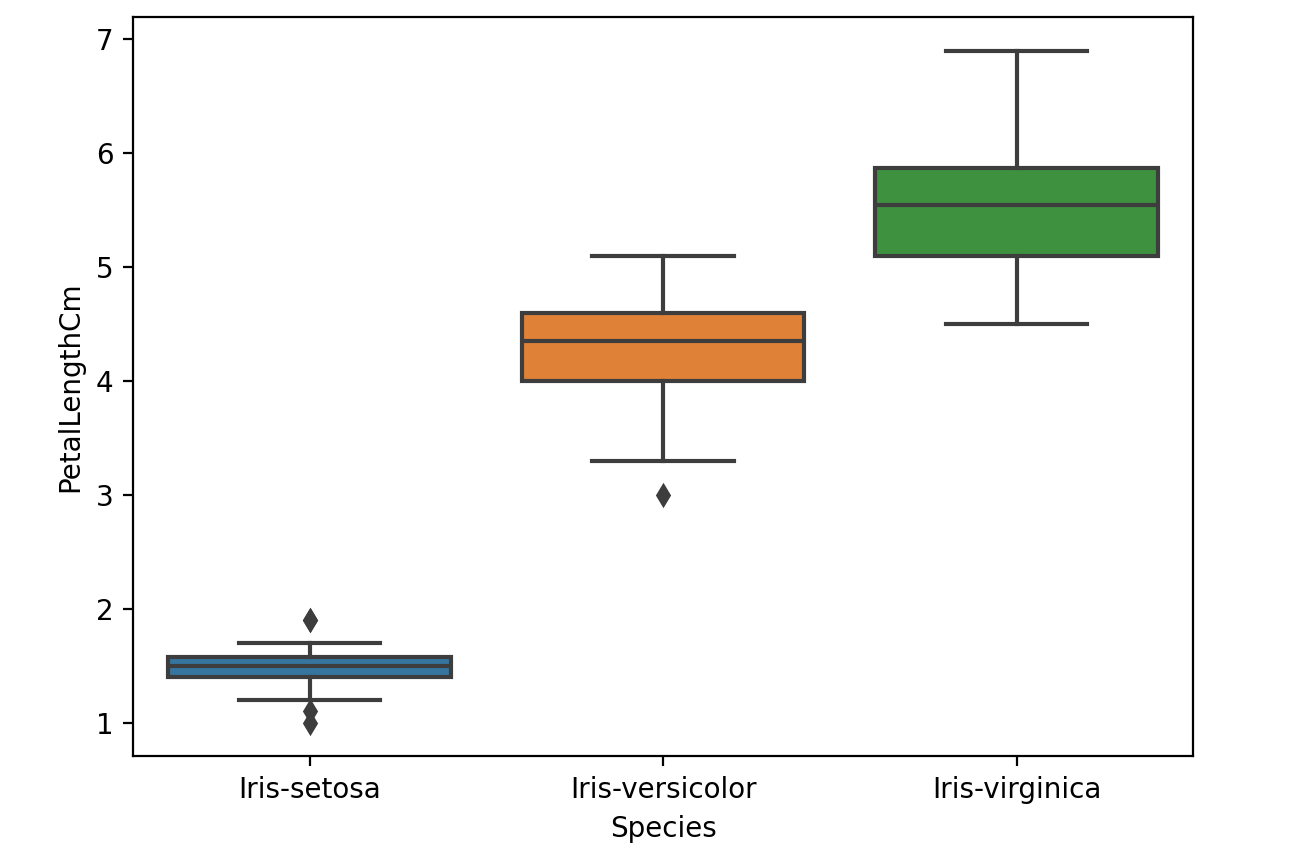
我们扩展该图的一种方法是在上面添加一层单独的点。我们将使用jitter=True
以便所有点都不会落在单个垂直线上。
1 | # One way we can extend this plot is adding a layer of individual points on top of |
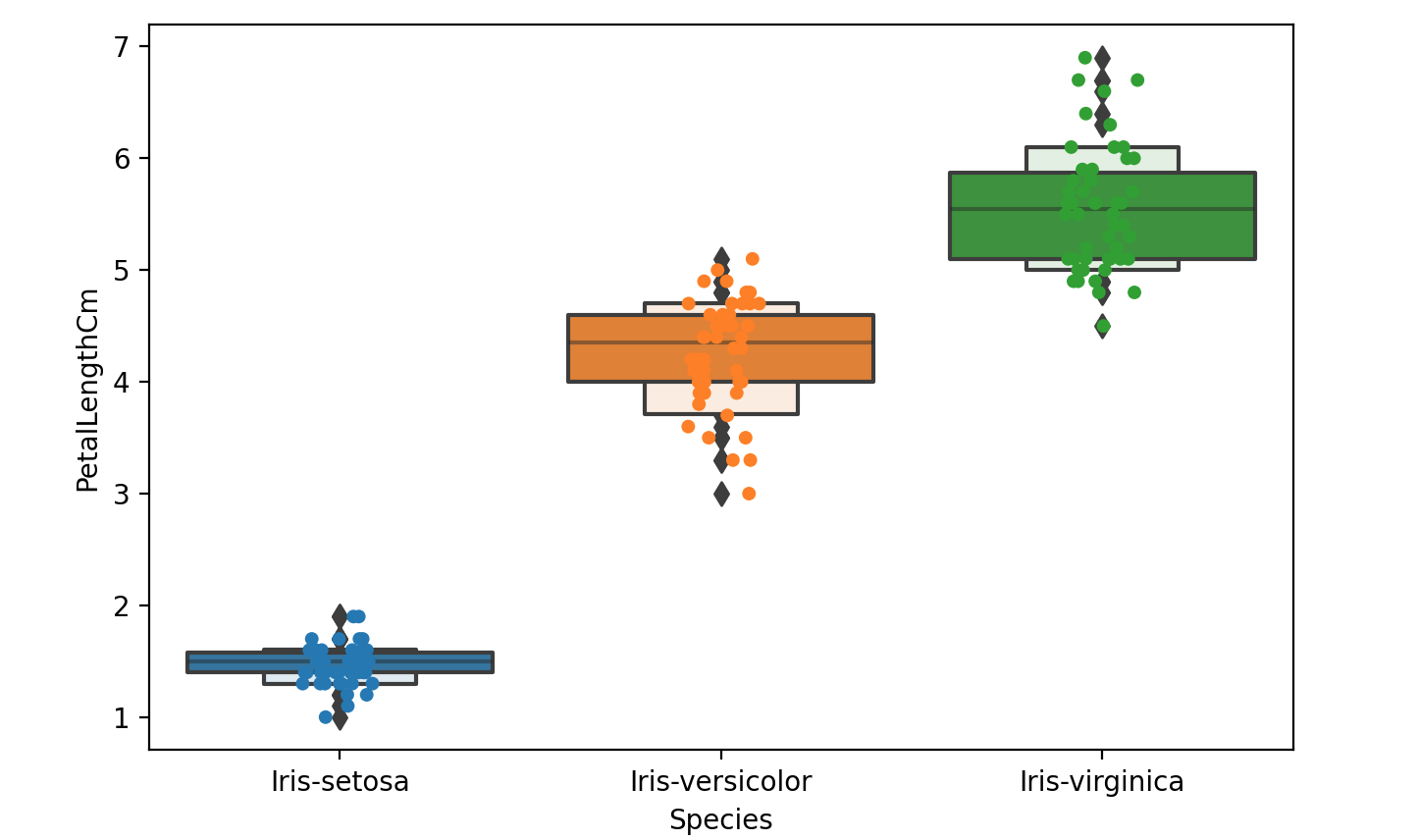
小提琴图结合了前两个图的优点并简化了它们在小提琴图中,数据的密集区域更厚,稀疏区域更薄。
1 | # A violin plot combines the benefits of the previous two plots and simplifies them |
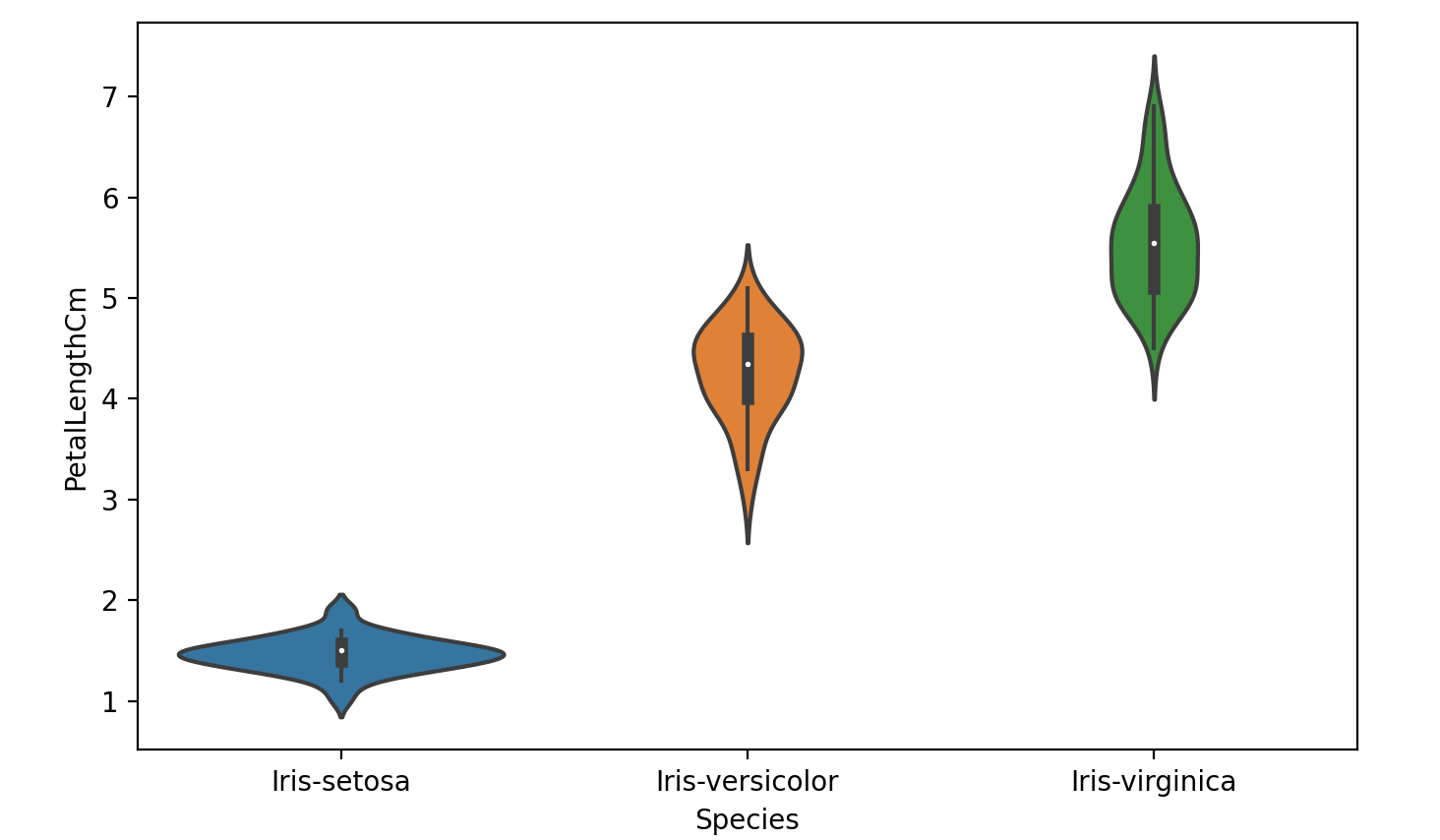
用于查看单变量关系的最后一个seaborn
图是kdeplot
,创建并可视化底层特征的核密度估计。
1 | # A final seaborn plot useful for looking at univariate relations is the kdeplot, |
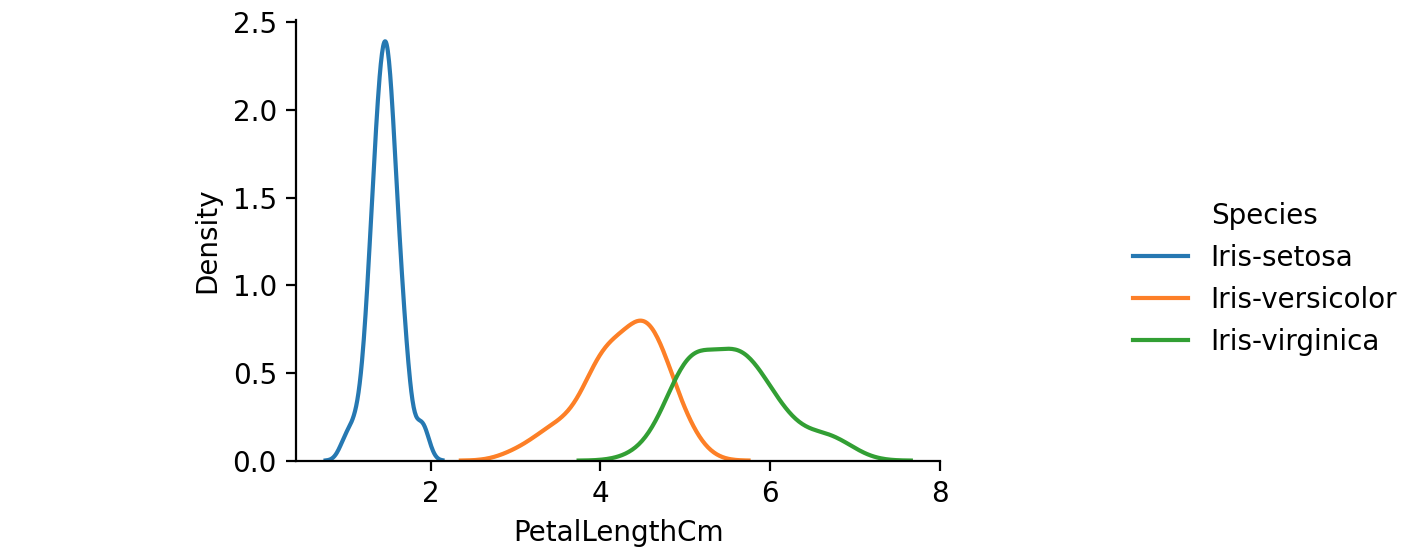
另一个有用的seaborn
图是pairplot
,它显示了每对特征之间的二元关系。从配对图中,我们可以看到,在所有特征组合中,Iris-setosa
物种都与其他两种物种分离。
1 | # Another useful seaborn plot is the pairplot, which shows the bivariate relation |
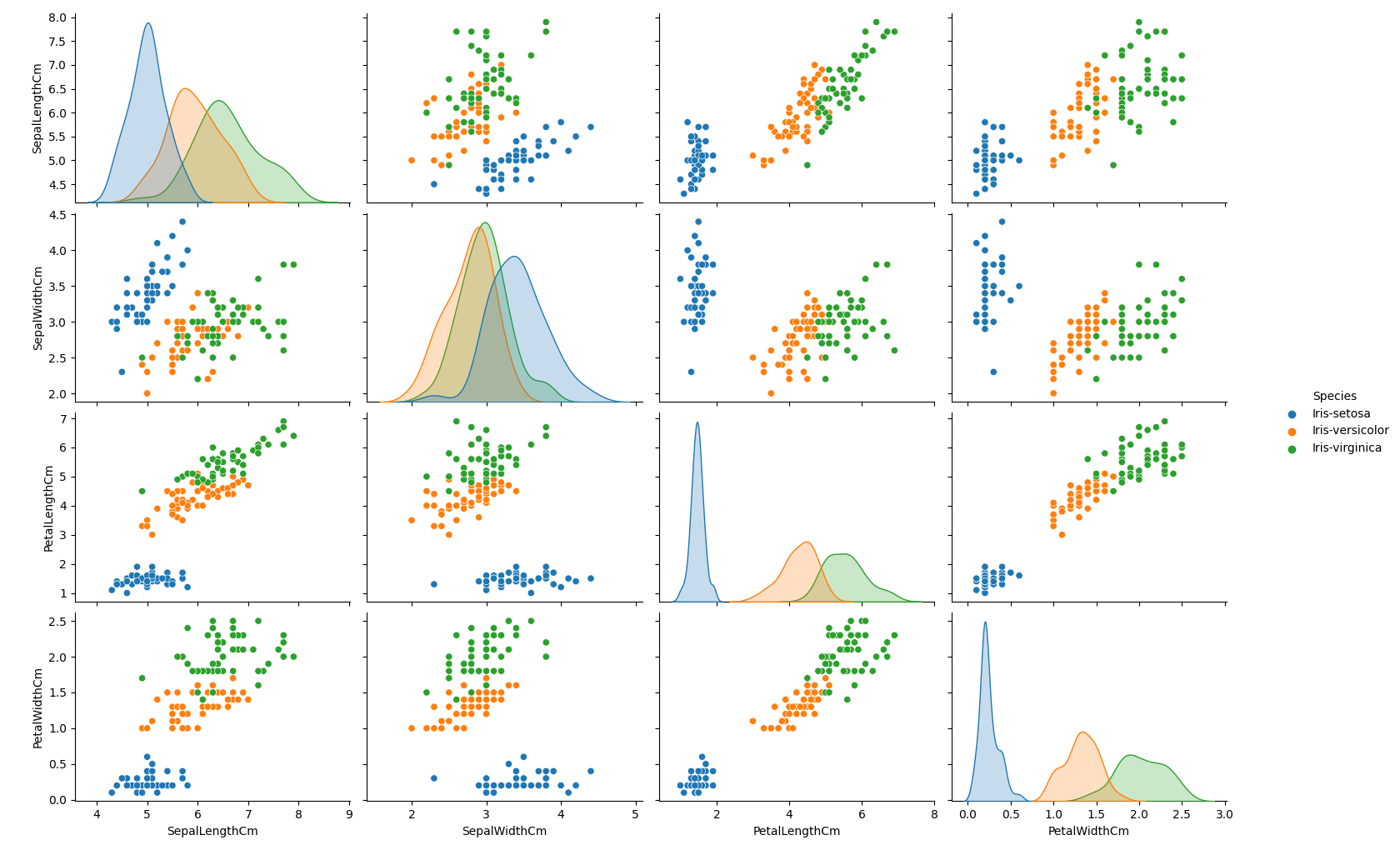
既然我们已经介绍了seaborn
,让我们回顾一下我们可以用Pandas
制作的一些图。我们可以用Pandas
快速制作一个箱线图,按物种划分每个特征。
1 | # Now that we've covered seaborn, let's go back to some of the ones we can make with Pandas |
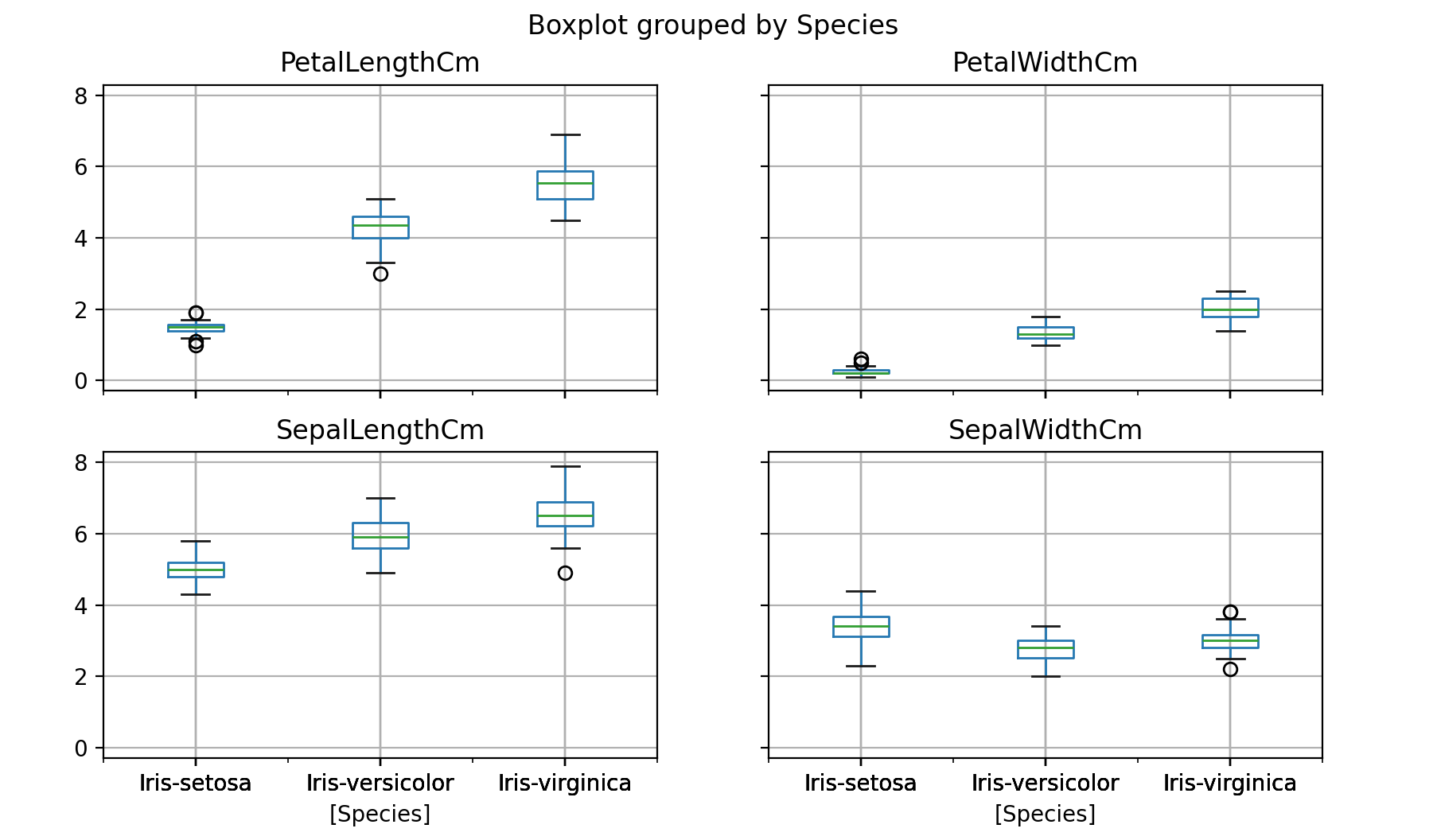
Pandas
中有一个更酷更复杂的技术叫做安德鲁斯曲线。安德鲁斯曲线涉及使用样本的属性作为傅里叶级数的系数。
1 | # One cool more sophisticated technique pandas has available is called Andrews Curves |
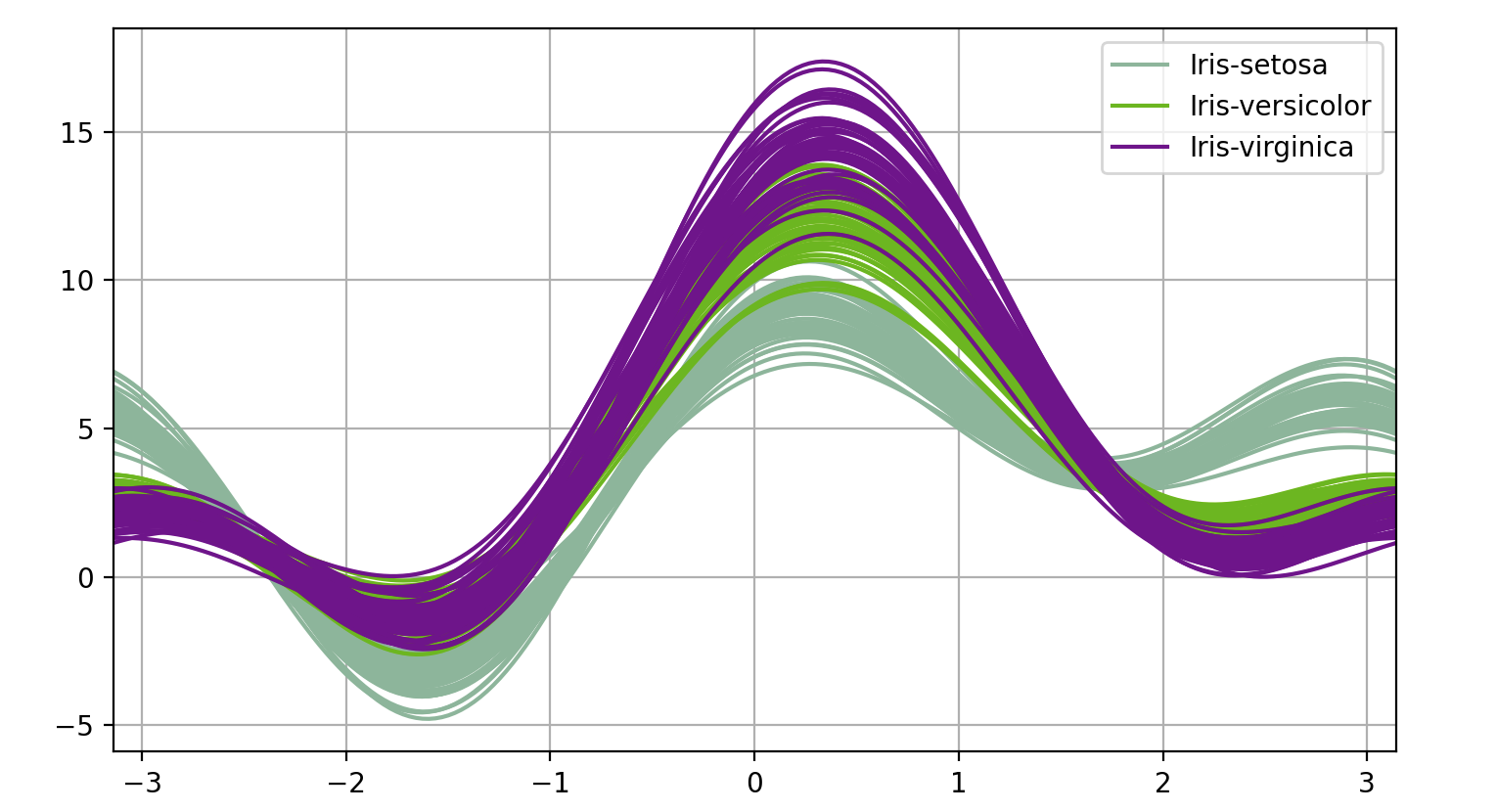
pandas
的另一种多元可视化技术是parallel_coordinates
平行坐标将每个特征绘制在单独的列上,然后绘制连接每个数据样本特征的线。
1 | # Another multivariate visualization technique pandas has is parallel_coordinates |
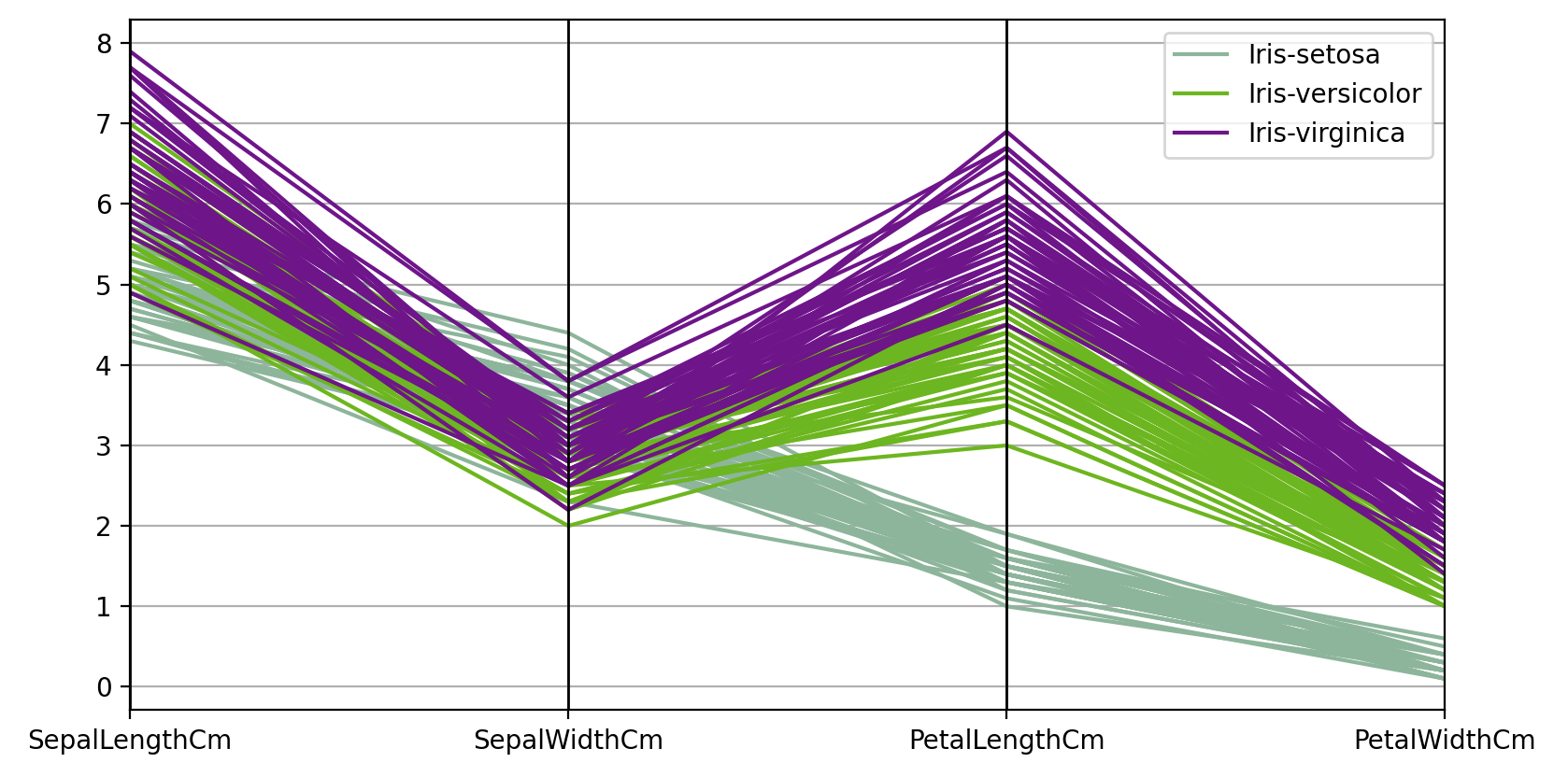
pandas
的最后一项多元可视化技术是radviz
。它将每个特征作为二维平面上的一个点,然后模拟将每个样本通过一个由该特征的相对值加权的弹簧连接到这些点。
1 | # A final multivariate visualization technique pandas has is radviz |
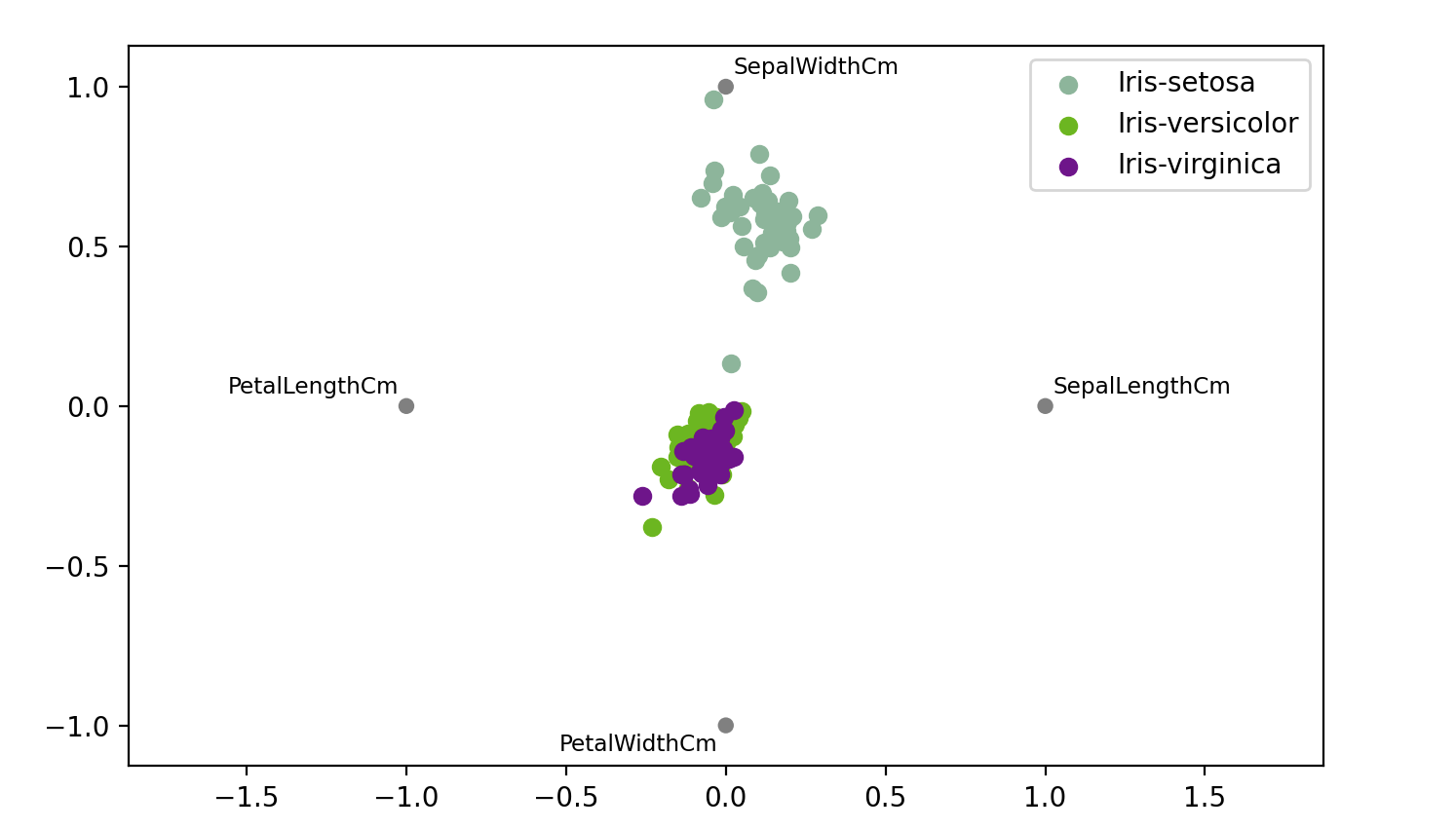